Java ElasticSearch implementation for window size more than 10k
Java ElasticSearch implementation with search_after feature
WEB DEVELOPMENT
PK
12/27/20232 min read
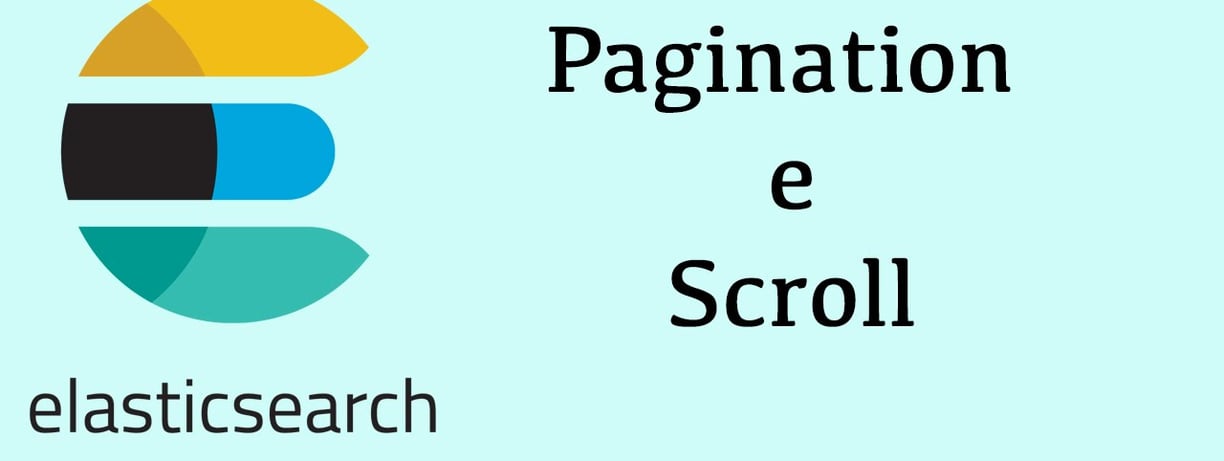
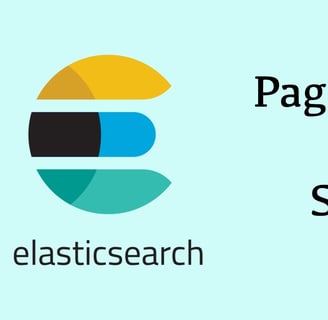
Introduction
Implementing search functionality using Spring Boot and Elasticsearch with the "search_after" feature involves a few steps. The "search_after" feature is used for efficient pagination of search results. It is often used in conjunction with sorting to navigate through large result sets.
Here's a basic guide on how you can implement this in a Spring Boot application:
Add Dependencies:
Ensure that you have the necessary dependencies in your pom.xml file:
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
</dependencies>
Configure Elasticsearch: Configure Elasticsearch properties in your application.properties or application.yml file:
spring.data.elasticsearch.cluster-name=my-cluster-name
spring.data.elasticsearch.cluster-nodes=localhost:9300
Adjust these properties based on your Elasticsearch configuration.
Create an Entity: Create an entity class representing your data model. Annotate it with @Document to mark it as an Elasticsearch document.
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
@Document(indexName = "your_index_name", type = "your_type")
public class YourEntity {
@Id
private String id;
// Other fields, getters, and setters
}
Repository Interface: Create a repository interface that extends ElasticsearchRepository:
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
public interface YourEntityRepository extends ElasticsearchRepository<YourEntity, String> {
// Custom query methods if needed
}
Service Class: Create a service class where you can implement the logic for searching with "search_after":
import org.elasticsearch.search.SearchHit;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate;
import org.springframework.data.elasticsearch.core.SearchHitCallback;
import org.springframework.data.elasticsearch.core.query.NativeSearchQuery;
import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder;
import org.springframework.data.elasticsearch.core.query.SearchQuery;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class YourEntityService {
@Autowired
private ElasticsearchRestTemplate elasticsearchRestTemplate;
@Autowired
private YourEntityRepository repository;
public List<YourEntity> searchWithSearchAfter(String searchAfterValue, int pageSize) {
SearchQuery searchQuery = new NativeSearchQueryBuilder()
.withQuery(/* your query criteria here */)
.withSort(/* your sorting criteria here */)
.withPageable(/* use PageRequest.of(0, pageSize) for the first page */)
.withSearchAfter(searchAfterValue) // Set the search_after value
.build();
return elasticsearchRestTemplate.search(searchQuery, YourEntity.class,
new SearchHitCallback<YourEntity>() {
@Override
public List<YourEntity> doWithSearchHits(SearchHit<YourEntity> searchHit) {
// Extract and return the list of entities
return List.of(searchHit.getContent());
}
});
}
}
Customize the withQuery, withSort, and other methods in NativeSearchQueryBuilder based on your search requirements.
Controller: Create a controller to expose the search functionality:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class YourEntityController {
@Autowired
private YourEntityService service;
@GetMapping("/search")
public List<YourEntity> searchWithSearchAfter(@RequestParam String searchAfterValue, @RequestParam int pageSize) {
return service.searchWithSearchAfter(searchAfterValue, pageSize);
}
}
This controller endpoint can be called with the searchAfterValue and pageSize parameters to retrieve the next set of results.
Remember to adapt these examples according to your specific requirements and use case. The provided code serves as a starting point, and you may need to make adjustments based on your application's needs.
Address
401 Kartik Kunj Sector 44 Noida, UP 201303